实验内容
- 编写一个简单的Java Applet程序,并在Java Applet中写两行文字:“这是一个Java Applet”和和“我改变了字体”。
- 练习
- 输出1-200之间的素数;
- 嵌套循环(for + while)实现1!+2!+3!+ … 9!即阶乘之和(如果能采用递归算法实现阶乘,再调用此函数实现求和,更妙);
- 尝试用applet编写程序实现摄氏温度和华氏温度的转换。
- 利用JOptionPane类编写一个计算电费的程序。
1. Java Applet基础
code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| package project2;
import java.applet.Applet; import java.awt.*;
public class MyFirstApplet extends Applet { public void paint(Graphics g){ g.setColor(Color.GREEN); g.drawString("这是一个Java Applet程序",1,15); g.setColor(Color.red); g.setFont(new Font("宋体",Font.BOLD,36)); g.drawString("我改变了字体",1,50); }
}
|
运行结果
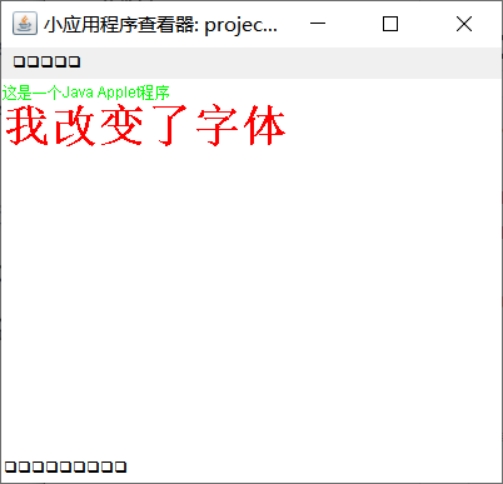
2. 练习
1. 程序中的主类如果不用public修饰,编译能通过吗?
可以通过编译。
2. 程序中的主类如果不用public修饰,程序能正确运行吗?
不能,运行报错:
加载: project2.MyFirstApplet不是公共的, 或者没有公共构造器。
如果一个编译单元(文件)中含有public的类,则文件名必须与类名一致,如果没有public类,则文件可以随意命名。 一个文件中,public的类可以有零个或一个,非public的类可以有零个或多个。Java程序是从一个public类的main函数开始执行的,(其实是main线程),就像C程序是从main( ) 函数开始执行一样。只能有一个public类是为了给类装载器提供方便。一个public 类只能定义在以它的类名为文件名的文件中。每个编译单元(文件)都只有一个public 类。因为每个编译单元都只能有一个公共接口,用public类来表现。该接口可以按照要求包含众多的支持包访问权限的类。如果有一个以上的public 类,编译器就会报错。 并且public类的名称必须与文件名相同(严格区分大小写)。当然一个编译单元内也可以没有public类。
3. 程序将paint方法误写成Paint,编译能通过么?
编译能通过。
4. 程序将paint方法误写成Paint,运行时能看到有关的输出信息吗?
不能,涉及到继承问题,子类MyFirstApplet继承了父类Applet的paint方法,在子类通过重写paint方法达到绘制输出信息的目的,如果将paint方法写成Paint方法,就无法达到目的。
补充
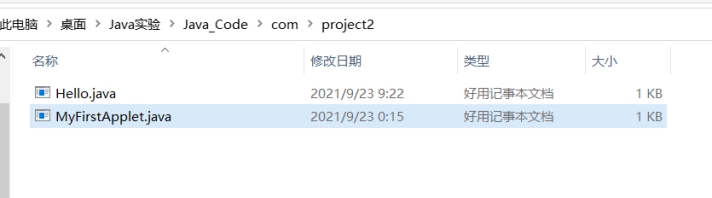
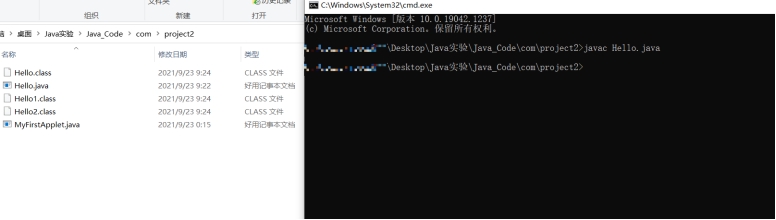
以上尝试在一个java文件中编写3个类,编译后会产生3个class文件。
说明java文件内有多少个类,编译后就会产生多少个class文件。
3. 输出1-200之间的素数
code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package project2;
public class Prime { public static void main(String[] args) { int sum = 0; for(int i = 2;i <= 200;i++){ if(prime(i)==true){ System.out.printf(i+"\t"); sum++; if (sum%5==0) System.out.println(); } } System.out.println(); System.out.println("1~200一共有"+sum+"个素数"); }
public static boolean prime(int n){ for(int j = 2; j <= n/2;j++) if (n%j==0){ return false; } return true; } }
|
运行结果
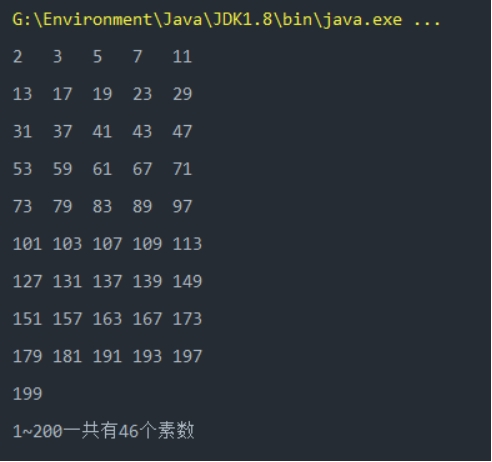
4. 嵌套循环(递归)
code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| package project2;
import java.util.Scanner;
public class Factorial { public static void main(String[] args) { int sum = 0; for(int i = 1;i <= 9;i++){ sum+=mux(i); } System.out.println("1!+2!+...+9! = "+sum); }
public static int mux(int n){ if(n==1) return 1; else return n*mux(n-1); } }
|
运行结果
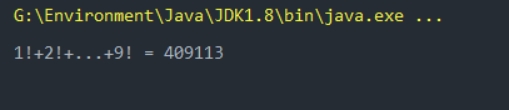
5. 摄氏度和华氏度的转换
code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80
| package project2;
import com.sun.xml.internal.ws.commons.xmlutil.Converter;
import javax.swing.*; import javax.swing.border.Border; import java.applet.Applet; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.image.ImageProducer;
public class TempTrans extends Applet implements ActionListener { String text1,text2; private static final long serialVersionUID = 1L; TextField temp1,temp2; Label lab1,lab2,lab3,lab4; JButton button1;
public void init(){ lab1 = new Label("摄氏温度"); lab2 = new Label("华氏温度"); lab3 = new Label("℃"); lab4 = new Label("℉"); temp1 = new TextField(10); temp2 = new TextField(10);
button1 = new JButton("转换"); button1.addActionListener(this); button1.setActionCommand("a");
add(lab1); add(temp1); add(lab3); add(lab2); add(temp2); add(lab4); add(button1, BorderLayout.PAGE_END); temp1.addActionListener(this); temp2.addActionListener(this); }
@Override public void actionPerformed(ActionEvent e) { if (e.getActionCommand().equals("a")) { if (!((temp1.getText().equals("")) && (temp2.getText().equals("")))) { if (temp1.getText().equals("") (temp1.getText().equals(text1))) { String fah = temp2.getText(); Double fah1 = Double.valueOf(fah).doubleValue(); Double cel2 = (fah1 - 32) / 1.8; float cel3 = (float) (Math.round(cel2 * 100)) / 100; temp1.setText(cel3 + ""); text1 = temp1.getText(); text2 = temp2.getText(); } else if (temp2.getText().equals("") temp2.getText().equals(text2)) { String cel = temp1.getText(); Double cel1 = Double.valueOf(cel).doubleValue(); Double fah2 = 1.8 * cel1 + 32; float fah3 = (float) (Math.round(fah2 * 100)) / 100; temp2.setText(fah3 + ""); text1 = temp1.getText(); text2 = temp2.getText(); } else if((!(temp1.getText().equals(text1)))&&(!(temp2.getText().equals(text2)))){ String cel = temp1.getText(); Double cel1 = Double.valueOf(cel).doubleValue(); Double fah2 = 1.8 * cel1 + 32; float fah3 = (float) (Math.round(fah2 * 100)) / 100; temp2.setText(fah3 + ""); text1 = temp1.getText(); text2 = temp2.getText(); } } } } }
|
该程序在实例的基础上修缮了以下bug:
- 当两边输入均为空的情况下控制台的报错
- 当要进行转换时,必须保证输出文本框为空
增加了以下功能:
- 当一边数值发生变化时,便会以变化的数值作为计算的数据,另一边则作为输出
- 当两边数同时变化时,以摄氏度的数值为准
运行结果
- 输入摄氏度,华氏度为空
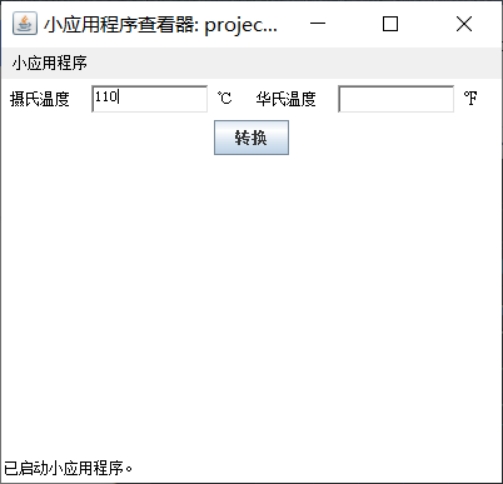
转换:
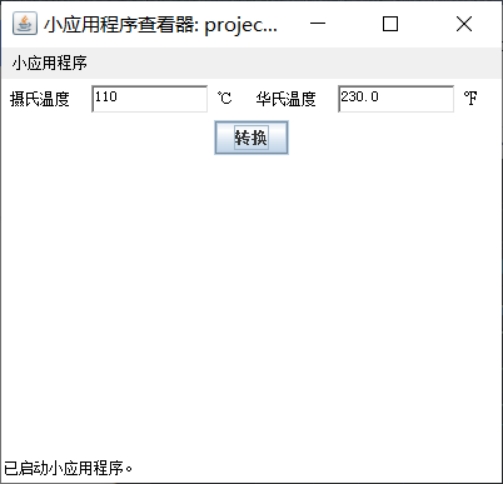
- 基于上次转换结果的基础上直接改变某一边的值且不用清除另一边的输出:
(1)将摄氏度值改为100
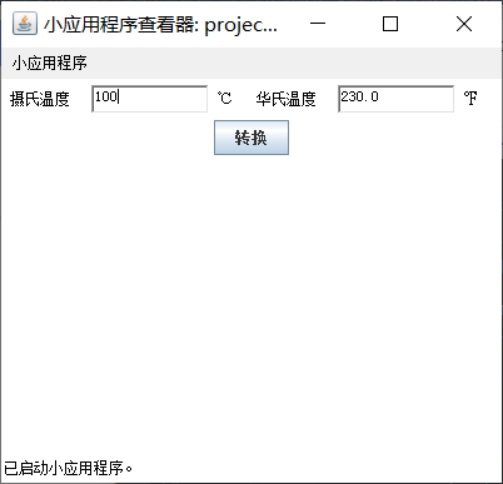
转换:

(2)将华氏度数值改变为0
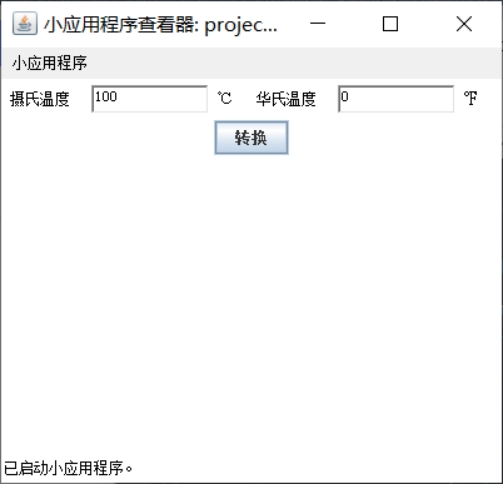
转换:
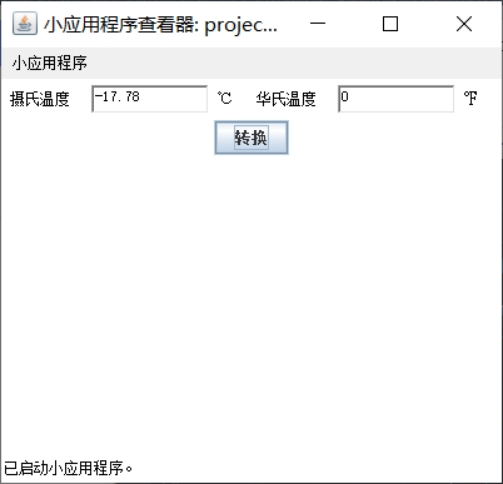
- 两边数值同时改变:
将两边数值均改为100

转换:
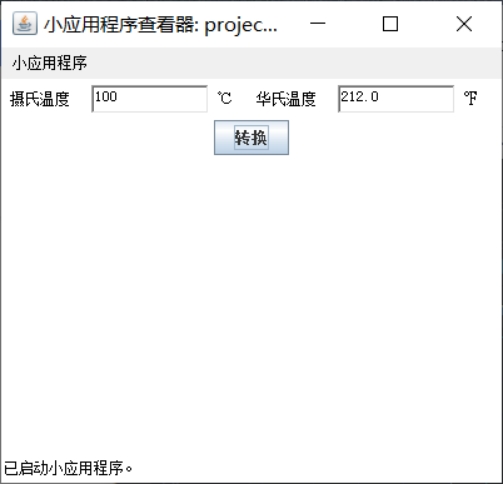
以摄氏度数值为准
6. 电费计算
code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| package project2;
import com.sun.xml.internal.ws.commons.xmlutil.Converter;
import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener;
public class ElectricCharge { public static void main(String[] args) { String str = JOptionPane.showInputDialog(null, "请输入您的实际使用电量(度):", "电量输入", 3); if (str != null){ float elec = Float.valueOf(str).floatValue(); float elecCharge = 0; if (elec <= 240) { elecCharge += elec * 0.55; } else if ((elec > 240) && (elec <= 540)) { elecCharge += 240 * 0.55 + (elec - 240) * 0.7; } else if (elec > 540) { elecCharge += 240 * 0.55 + 300 * 0.7 + (elec - 540) * 0.95; } String result = String.format("%.2f", elecCharge);
JOptionPane.showMessageDialog(null, "您的电费金额为" + result + "元", "电费总额", JOptionPane.PLAIN_MESSAGE); } } }
|
运行结果
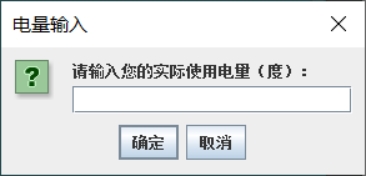
提示输入使用电量
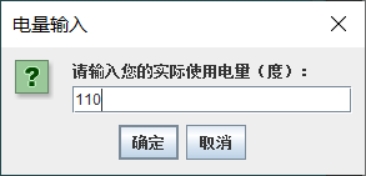
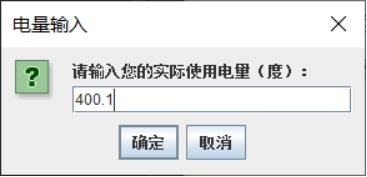
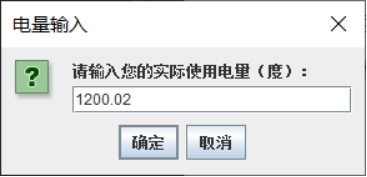
确定后弹出消息对话框:
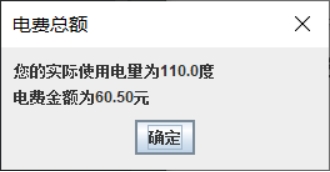
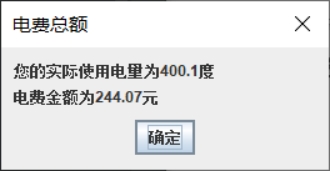
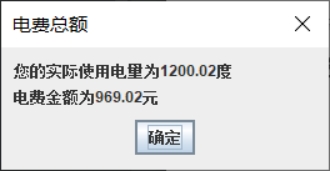
实验心得
通过摄氏度和华氏度转换的程序,我发觉debug是一种不断追求完美的乐趣。一个程序给到不同的人大体实现思路都一样,就要看谁实现的功能更强大,bug更少,更符合人性化的设计。这也是GUI和UI界面设计的乐趣所在。